Project: AddressBook - Level 4
AddressBook - Level 4 is a desktop address book application used for teaching Software Engineering principles. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 6 kLoC.
Code contributed: [Functional code] [Test code] {give links to collated code files}
Listing all persons : (l)list
You want to view all your contacts in your contact list.
Format: (l)list
This will list out all of your contacts in alphabetical order.
Example:
-
list
Contacts will be sorted by their names (increment). |
Locating persons by their tag : (ft)findtag
Finds persons whose corresponding tags contain any of the given keywords and lists them out.
Format: (ft)findtag KEYWORD [MORE_KEYWORDS]…
Examples:
-
findtag family
Returns any person that contains afamily
tag -
findtag family colleagues
Returns any person that contains the tagsfamily
andcolleagues
Emailing a person : (em)email
Emails a person (identified by the index number used in the last listing) to email that person.
Format: (em)email INDEX
Examples:
-
list
email 2
Emails the 2nd person in the address book.
Directions to a person’s address : (gm)gmap
Opens Google Maps in default browser with a person’s (identified by the index number used in the last
listing) address in the Destination.
Format: (gm)gmap INDEX
Examples:
-
list
gmap 2
The address of the 2nd person in the address book will be in the Destination field in Google Maps.
Finding a contact on Facebook : (fb)facebook
Searches for a person’s (identified by the index number used in the last listing) name on Facebook.
Format: (fb)facebook INDEX
Examples:
-
list
facebook 2
The name of the 2nd person in the address book will be searched on Facebook.
Justification
Listing all persons:
There is a need to have the contacts sorted by default as this would allow users to be able to look through
a more organized contact list by default, rather than one without order.
Locating persons by their tag:
This is necessary for better organization as it will reduce the time users need to spend scrolling through
the entire list to look for contacts from a particular social group. This adds on to the convenient factor
of the application.
Emailing a person:
This command allows users to be able to email their contacts hassle-free as they do not need to open their
email, compose a new email and copy their contact’s email inside. Instead, after typing in the command line,
the default mail app will appear with the email of the contact already inside.
Google Map directions to a person’s address:
This provides a shortcut to Google Maps using the default browser with the Destination filled up with
a contact’s address. This will allow users to easily get directions to their contact’s house for when
users are visiting their friends or family. This saves users the of mundane task of having to manually open Google Maps
and typing in their contact’s address into the Destination field.
Searching for a contact on Facebook:
Users can easily search for their contacts on Facebook. As most people use social media as a way to keep up with
their friends' or family’s lives, they often want to add them of platforms such as Facebook. This feature offers a
convenient way for users to search for any of their contacts on Facebook to add them.
Calendar
This allows users to be able to easily check their events with a monthly view to see what day of the week it is and be
able to clearly see in how many weeks time is the event happening. Also, users can use it to look at the calendar
conveniently without having to open any external applications. A calendar is often used when doing scheduling or
planning and is thus useful when placed with the events.
Emailing mechanism
The automatic opening of email on the user’s desktop is facilitated mainly by the Desktop
class which allows the Java
application to launch the default mail application registered inside the user’s native desktop to handle the email URI
.
Calendar mechanism
An in-built calendar is added to the panel beside the list of events. This calendar shows a monthly view of the current month.
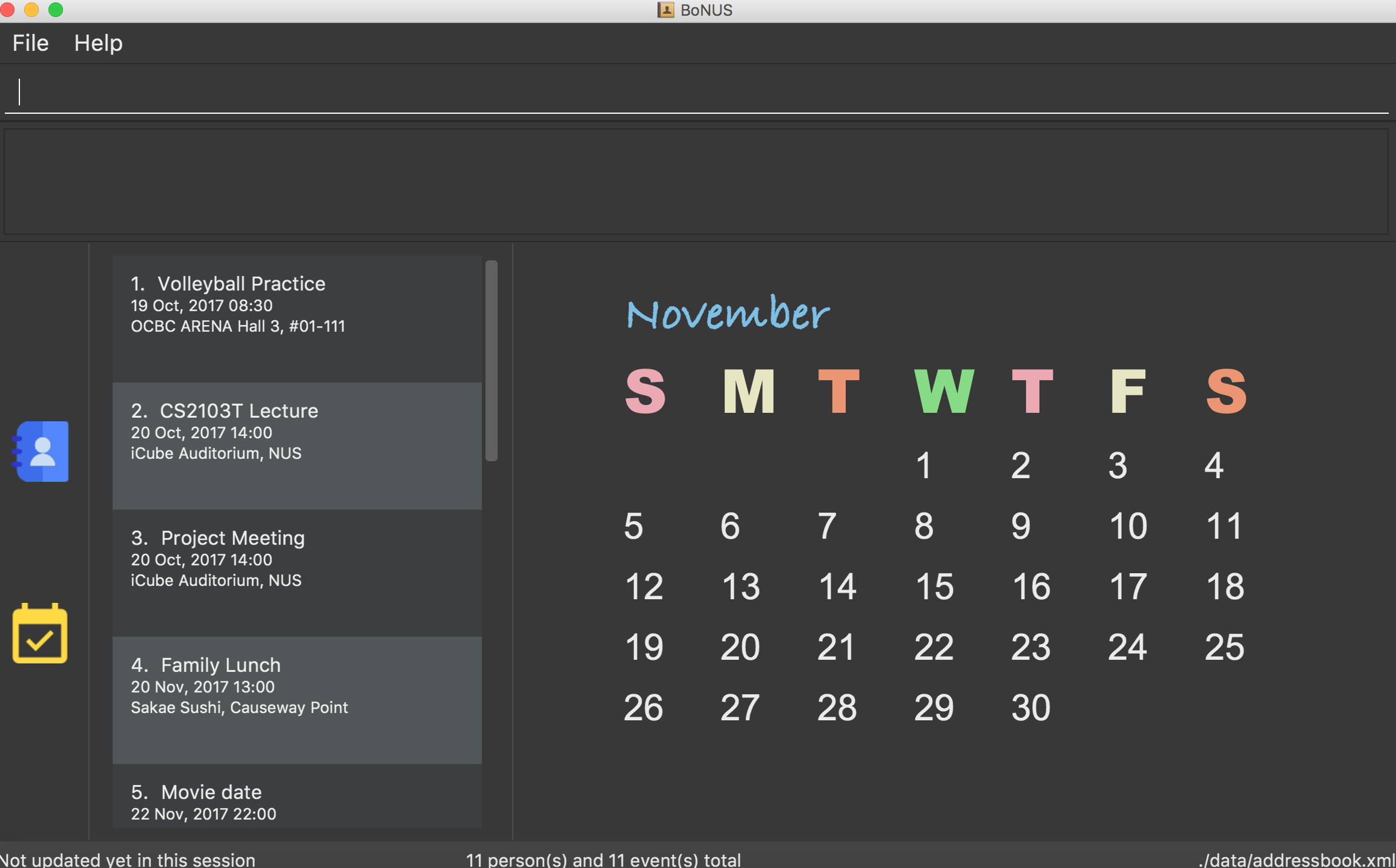
Figure 4.10.1 : Calendar for month of November 2017
This is useful for users to be able to compare their list of events in the calendar. It can help users to check what day of the week their event is happening on. This is especially useful for NUS students as there is a fixed daily schedule and it would be easy to check what day it falls on to ensure their availability for the event. Other usages include having to see what dates lie on a particular day for students to plan weekly events. All in all, it provides a convenient way for students to have access to a calendar without having to open any external applications.
Design Considerations
Aspect: How to display the individual dates.
Alternative 1 (current choice): Use a GridPane to design the table in XML. Include a class to calculate dates to be
shown, before populating individual cells in GridPane using data from class.
Pros: This method makes use of JavaFX, possibly allowing us to improve the aesthetic design in the future. It also
allows individual objects to be created for individual cells. This could allow us to write more functionality such
as showing number of events per day to dynamic colour changes in the future. The monthDateBuilder
method provides an
accurate graphical representation of the days in a monthly calendar.
Cons: The 42 cell design is fairly cumbersome, requiring the initialization of at least 42 cells. The class is limited
in a way that it must have 42 cells, thus sometimes many cells are left unused, and there is a wastage of memory.
Alternative 2: Use JTable to display individual dates.
Pros: A ready to use API that can allow easy building of table showing dates. It is also a powerful tool with many
functions.
Cons: JTable is a UI element from a different UI design model, and cannot make use of advantages brought about by the
new advantages from JavaFX, such as the node capability of JavaFX and certain aesthetic modifications. Many of the
JTable functions might not be used.
Implementation Outline
-
Create a
EventCalendar
class that contains the month that will be shown, containing all the necessary information required for a monthly calendar (i.e. dates, name of month). -
Create a
monthDateBuilder
class that will be used to set the current month and year of the calendar. It will also be arranging the dates in a way that will correspond to which day of the week it belongs to. -
Use
Calendar
class to set the current year and month and store in an array (i.e.monthYearArray
) inmonthDateBuilder
. Also, useCalendar
class to set the number of days in the current month and the day that the first date of the month falls on (i.e. set usingsetMonthAnchors
method). These details are required to build the monthly view. -
There are a maximum of 42 cells to fill up in every month. Create a method (i.e.
buildMonthArray
method) to store the position of the dates in. Use an algorithm to store all the dates before the first date of the month as a blank, fill up the subsequent dates using the value of the first day of the month and their cell number, and the leftover dates will continue to be blanks until the 42nd cell.
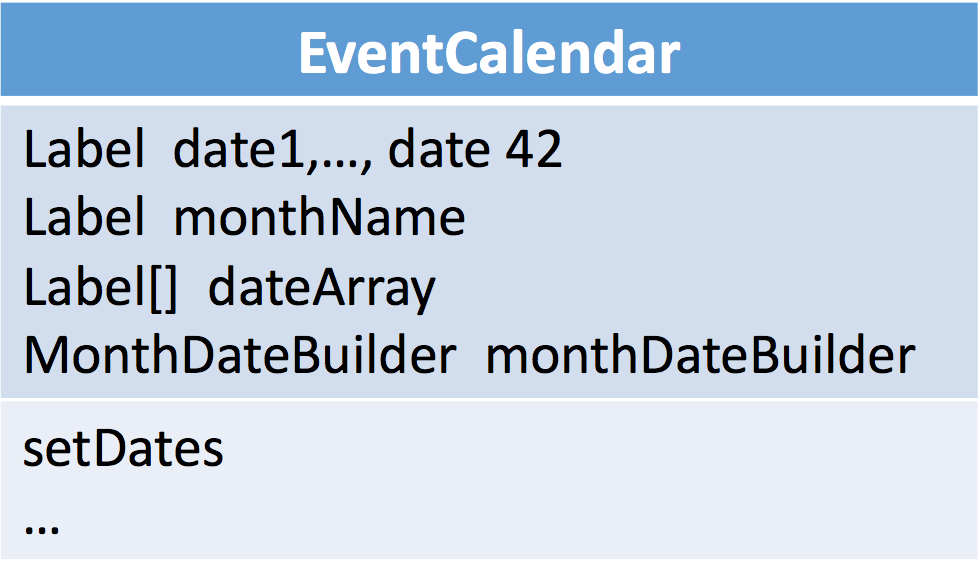
Figure 4.10.1.1 : Class Diagram for `EventCalendar`
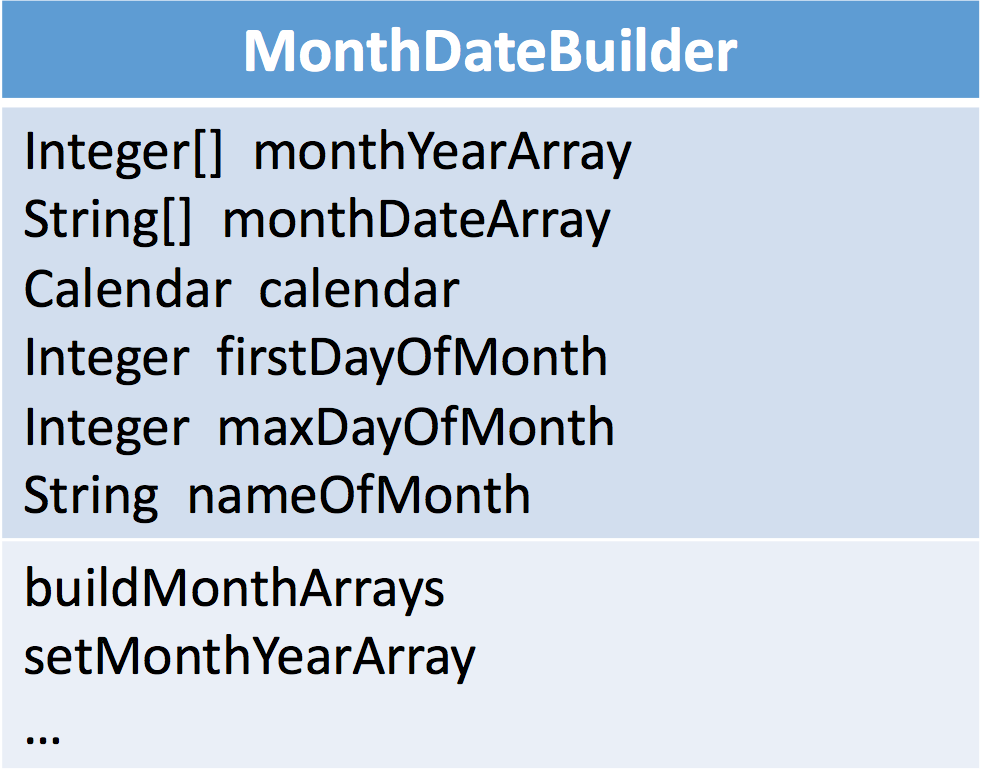
Figure 4.10.1.2 : Class Diagram for `MonthDateBuilder`
Enhancement Proposed: Add Subject and more emails via command line, More Interactive Calendar
Email
This will make it more convenient to email multiple people at once.
Calendar
Future plans include highlighting today’s date, having weekly or even yearly views, being able to view any month
instead of just the current month. Future enhancements also include integrating the list of events into the calendar.